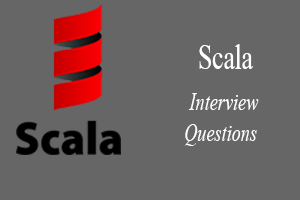
Scala Interview Questions
1) What is Scala?
Scala is a general-purpose programming language. It supports object-oriented, functional and imperative programming approaches. It is a strong static type language. In Scala, everything is an object whether it is a function or a number. It was designed by Martin Odersky in 2004.
Scala Program Example
For more information: Click here.
2) What are the features of Scala?
There are following features in Scala:
- Type inference: In Scala, you don’t require to mention data type and function return type explicitly.
- Singleton object: Scala uses a singleton object, which is essentially class with only one object in the source file.
- Immutability: Scala uses immutability concept. Immutable data helps to manage concurrency control which requires managing data.
- Lazy computation: In Scala, computation is lazy by default. You can declare a lazy variable by using the lazy keyword. It is used to increase performance.
- Case classes and Pattern matching: In Scala, case classes support pattern matching. So, you can write more logical code.
- Concurrency control: Scala provides a standard library which includes the actor model. You can write concurrency code by using the actor.
- String interpolation: In Scala, string interpolation allows users to embed variable references directly in processed string literals.
- Higher order function: In Scala, higher order function allows you to create function composition, lambda function or anonymous function, etc.
- Traits: A trait is like an interface with partial implementation. In Scala, the trait is a collection of abstract and non-abstract methods.
- Rich set of collection: Scala provides a rich set of collection library. It contains classes and traits to collect data. These collections can be mutable or immutable.
For more information: Click here.
3) What are the Data Types in Scala?
Data types in Scala are much similar to Java regarding their storage, length, except that in Scala there is no concept of primitive data types every type is an object and starts with capital letter. A table of data types is given below.
Data Types in Scala
Data Type | Default Value | Size |
---|---|---|
Boolean | False | True or false |
Byte | 0 | 8 bit signed value (-27 to 27-1) |
Short | 0 | 16 bit signed value(-215 to 215-1) |
Char | ‘u0000’ | 16 bit unsigned Unicode character(0 to 216-1) |
Int | 0 | 32 bit signed value(-231 to 231-1) |
Long | 0L | 64 bit signed value(-263 to 263-1) |
Float | 0.0F | 32 bit IEEE 754 single-precision float |
Double | 0.0D | 64 bit IEEE 754 double-precision float |
String | Null | A sequence of characters |
For more information: Click here.
4) What is pattern matching?
Pattern matching is a feature of Scala. It works same as switch case in other languages. It matches the best case available in the pattern.
Example
For more information: Click here.
5) What is for-comprehension in Scala?
In Scala, for loop is known as for-comprehensions. It can be used to iterate, filter and return an iterated collection. The for-comprehension looks a bit like a for-loop in imperative languages, except that it constructs a list of the results of all iterations.
Example
For more information: Click here.
6) What is the breakable method in Scala?
In Scala, there is no break statement, but you can do it by using break method and importing Scala.util.control.Breaks._ package. It can break your code.
Example
For more information: Click here.
7) How to declare a function in Scala?
In Scala, functions are first-class values. You can store function value, pass a function as an argument and return function as a value from other function. You can create a function by using the def keyword. You must mention return type of parameters while defining a function and return type of a function is optional. If you don’t specify the return type of a function, default return type is Unit.
Scala Function Declaration Syntax
For more information: Click here.
8) Why do we use =(equal) operator in Scala function?
You can create a function with or without = (equal) operator. If you use it, the function will return value. If you don’t use it, your function will not return anything and will work like the subroutine.
Example
For more information: Click here.
9) What is the Function parameter with a default value in Scala?
Scala provides a feature to assign default values to function parameters. It helps in the scenario when you don’t pass value during function calls. It uses default values of parameters.
Example
For more information: Click here.
10) What is a function named parameter in Scala?
In Scala function, you can specify the names of parameters while calling the function. You can pass named parameters in any order and can also pass values only.
Example
For more information: Click here.
11) What is a higher order function in Scala?
Higher order function is a function that either takes a function as an argument or returns a function. In other words, we can say a function which works with function is called a higher-order function.
Example
For more information: Click here.
12) What is function composition in Scala?
In Scala, functions can be composed from other functions. It is a process of composing in which a function represents the application of two composed functions.
Example
For more information: Click here.
13) What is Anonymous (lambda) Function in Scala?
An anonymous function is a function that has no name but works as a function. It is good to create an anonymous function when you don’t want to reuse it later. You can create anonymous function either by using ⇒ (rocket) or _ (underscore) wildcard in Scala.
Example
For more information: Click here.
14) What is a multiline expression in Scala?
Expressions those are written in multiple lines are called multiline expression. In Scala, be careful while using multiline expressions.
Example
The above program does not evaluate the complete expression and return b here.
For more information: Click here.
15) What is function currying in Scala?
In Scala, the method may have multiple parameter lists. When a method is called with a fewer number of parameter lists, this will yield a function taking the missing parameter lists as its arguments.
Example
For more information: Click here.
16) What is a nexted function in Scala?
In Scala, you can define the function of variable length parameters. It allows you to pass any number of arguments at the time of calling the function.
Example
For more information: Click here.
17) What is an object in Scala?
The object is a real-world entity. It contains state and behavior. Laptop, car, cell phone are the real world objects. An object typically has two characteristics:
1) State: data values of an object are known as its state.
2) Behavior: functionality that an object performs is known as its behavior.
The object in Scala is an instance of a class. It is also known as runtime entity.
For more information: Click here.
18) What is a class in Scala?
The class is a template or a blueprint. It is also known as a collection of objects of similar type.
In Scala, a class can contain:
- Data member
- Member method
- Constructor
- Block
- Nested class
- Superclass information, etc.
Example
For more information: Click here.
19) What is an anonymous object in Scala?
In Scala, you can create an anonymous object. An object which has no reference name is called an anonymous object. It is good to create an anonymous object when you don’t want to reuse it further.
Example
For more information: Click here.
20) What is a constructor in Scala?
In Scala, the constructor is not a special method. Scala provides primary and any number of auxiliary constructors. It is also known as default constructor.
In Scala, if you don’t specify a primary constructor, the compiler creates a default primary constructor. All the statements of the class body treated as part of the constructor.
Scala Primary Constructor Example
For more information: Click here.
21) What is method overloading in Scala?
Scala provides method overloading feature which allows us to define methods of the same name but having different parameters or data types. It helps to optimize code. You can achieve method overloading either by using different parameter list or different types of parameters.
Example
For more information: Click here.
22) What is this in Scala?
In Scala, this is a keyword and used to refer a current object. You can call instance variables, methods, constructors by using this keyword.
Example
For more information: Click here.
23) What is Inheritance?
Inheritance is an object-oriented concept which is used to reusability of code. You can achieve inheritance by using extends keyword. To achieve inheritance, a class must extend to other class. A class which is extended called super or parent class. A class which extends class is called derived or base class.
Example
For more information: Click here.
24) What is method overriding in Scala?
When a subclass has the same name method as defined in the parent class, it is known as method overriding. When subclass wants to provide a specific implementation for the method defined in the parent class, it overrides a method from the parent class.
In Scala, you must use either override keyword or override annotation to override methods from the parent class.
Example
For more information: Click here.
25) What is final in Scala?
Final keyword in Scala is used to prevent inheritance of super class members into the derived class. You can declare the final variable, method, and class also.
Scala Final Variable Example
For more information: Click here.
26) What is the final class in Scala?
In Scala, you can create a final class by using the final keyword. A final class can’t be inherited. If you make a class final, it can’t be extended further.
Scala Final Class Example
For more information: Click here.
27) What is an abstract class in Scala?
A class which is declared with the abstract keyword is known as an abstract class. An abstract class can have abstract methods and non-abstract methods as well. An abstract class is used to achieve abstraction.
Example
For more information: Click here.
28) What is Scala Trait?
A trait is like an interface with partial implementation. In Scala, the trait is a collection of abstract and non-abstract methods. You can create a trait that can have all abstract methods or some abstract and some non-abstract methods.
Example
For more information: Click here.
29) What is a trait mixins in Scala?
In Scala, “trait mixins” means you can extend any number of traits with a class or abstract class. You can extend only traits or combination of traits and class or traits and abstract class.
It is necessary to maintain the order of mixins otherwise compiler throws an error.
Example
For more information: Click here.
30) What is the access modifier in Scala?
Access modifier is used to define accessibility of data and our code to the outside world. You can apply accessibly to class, trait, data member, member method, and constructor, etc. Scala provides the least accessibility to access to all. You can apply any access modifier to your code according to your requirement.
In Scala, there are only three types of access modifiers.
- No modifier
- Protected
- Private
For more information: Click here
31) What is an array in Scala?
In Scala, the array is a combination of mutable values. It is an index based data structure. It starts from 0 index to n-1 where n is the length of the array.
Scala arrays can be generic. It means, you can have an Array[T], where T is a type parameter or abstract type. Scala arrays are compatible with Scala sequences – you can pass an Array[T] where a Seq[T] is required. Scala arrays also support all the sequence operations.
Example
For more information: Click here.
32) What is an ofDim method in Scala?
Scala provides an ofDim method to create a multidimensional array. The multidimensional array is an array which stores data in matrix form. You can create from two dimensional to three, four and many more dimensional array according to your need.
Example
For more information: Click here.
33) What is String in Scala?
In Scala, the string is a combination of characters, or we can say it is a sequence of characters. It is index-based data structure and uses a linear approach to store data into memory. The string is immutable in Scala like java.
Example
For more information: Click here.
34) What is string interpolation in Scala?
Starting in Scala 2.10.0, Scala offers a new mechanism to create strings from your data. It is called string interpolation. String interpolation allows users to embed variable references directly in processed string literals. Scala provides three string interpolation methods: s, f and raw.
Example
For more information: Click here.
35) What does s method in Scala String interpolation?
The s method of string interpolation allows us to pass a variable in the string object. You don’t need to use the + operator to format your output string. This variable is evaluated by the compiler and replaced by value.
Example
For more information: Click here.
36) What does f method in Scala String interpolation?
The f method is used to format your string output. It is like printf function of C language which is used to produce formatted output. You can pass your variables of any type in the print function.
Example
For more information: Click here.
37) What does raw method in Scala String interpolation?
The raw method of string interpolation is used to produce a raw string. It does not interpret special char present in the string.
Example
For more information: Click here.
38) What is exception handling in Scala?
Exception handling is a mechanism which is used to handle abnormal conditions. You can also avoid termination of your program unexpectedly.
Scala makes “checked vs. unchecked” very simple. It doesn’t have checked exceptions. All exceptions are unchecked in Scala, even SQLException, and IOException.
Example
For more information: Click here.
39) What is try catch in Scala?
Scala provides try and catch block to handle the exception. The try block is used to enclose suspect code. The catch block is used to handle exception occurred in the try block. You can have any number of the try-catch block in your program according to need.
Example
In this example, we have two cases in our catch handler. The first case will handle only arithmetic type exception. The second case has a Throwable class which is a super-class in the exception hierarchy. The second case can handle any type of exception in your program. Sometimes when you don’t know about the type of exception, you can use super-class.
For more information: Click here.
40) What is finally in Scala?
The finally block is used to release resources during exception. Resources may be a file, network connection, database connection, etc. The finally block executes guaranteed.
Example
For more information: Click here.
41) What is throw in Scala?
You can throw an exception explicitly in your code. Scala provides throw keyword to throw an exception. The throw keyword mainly used to throw a custom exception.
Example
For more information: Click here.
42) What is exception propagation in Scala?
In Scala, you can propagate the exception in calling chain. When an exception occurs in any function, it looks for the handler. If handler not available there, it forwards to caller method and looks for handler there. If handler presents there, handler catch that exception. If the handler does not present, it moves to next caller method in calling chain. This whole process is known as exception propagation.
43) What are throws in Scala?
Scala provides throws keyword for declaring the exception. You can declare an exception with method definition. It provides information to the caller function that this method may throw this exception. It helps to caller function to handle and enclose that code in a try-catch block to avoid abnormal termination of the program. In Scala, you can either use throws keyword or throws annotation to declare the exception.
Example
For more information: Click here.
44) What is a custom exception in Scala?
In Scala, you can create your exception. It is also known as custom exceptions. You must extend Exception class to while declaring custom exception class. You can create your message in custom class.
Example
For more information: Click here.
45) What is a collection in Scala?
Scala provides a rich set of collection library. It contains classes and traits to collect data. These collections can be mutable or immutable. You can use them according to your requirement.
For more information: Click here.
46) What is traversable in Scala collection?
It is a trait and used to traverse collection elements. It is a base trait for all Scala collections. It contains the methods which are common to all collections.
For more information: Click here.
47) What does Set in Scala collection?
It is used to store unique elements in the set. It does not maintain any order for storing elements. You can apply various operations on them. It is defined in the Scala.collection.immutable package.
Example
In this example, we have created a set. You can create an empty set also. Let’s see how to create a set.
for more information: Click here.
48) What does SortedSet in Scala collection?
In Scala, SortedSet extends Set trait and provides sorted set elements. It is useful when you want sorted elements in the Set collection. You can sort integer values and string as well.
It is a trait, and you can apply all the methods defined in the traversable trait and Set trait.
Example
for more information: Click here.
49) What is HashSet in Scala collection?
HashSet is a sealed class. It extends AbstractSet and immutable Set trait. It uses hash code to store elements. It neither maintains insertion order nor sorts the elements.
Example
For more information: Click here.
50) What is BitSet in Scala?
Bitsets are sets of non-negative integers which are represented as variable-size arrays of bits packed into 64-bit words. The largest number stored in it determines the memory footprint of a bitset. It extends Set trait.
Example
For more information: Click here.
51) What is ListSet in Scala collection?
In Scala, ListSet class implements immutable sets using a list-based data structure. In ListSet class elements are stored internally in a reversed insertion order, which means the newest element is at the head of the list. This collection is suitable only for a small number of elements. It maintains insertion order.
Example
For more information: Click here.
52) What is Seq in Scala collection?
Seq is a trait which represents indexed sequences that are guaranteed immutable. You can access elements by using their indexes. It maintains insertion order of elements.
Sequences support many methods to find occurrences of elements or subsequences. It returns a list.
Example
For more information: Click here.
53) What is Vector in Scala collection?
Vector is a general-purpose, immutable data structure. It provides random access of elements. It is suitable for a large collection of elements.
It extends an abstract class AbstractSeq and IndexedSeq trait.
Example
For more information: Click here.
54) What is List in Scala Collection?
The List is used to store ordered elements. It extends LinearSeq trait. It is a class for immutable linked lists. This class is useful for last-in-first-out (LIFO), stack-like access patterns. It maintains order, can contain duplicates elements.
Example
For more information: Click here.
55) What is the Queue in the Scala Collection?
Queue implements a data structure that allows inserting and retrieving elements in a first-in-first-out (FIFO) manner.
In Scala, Queue is implemented as a pair of lists. One is used to insert the elements and second to contain deleted elements. Elements are added to the first list and removed from the second list.
Example
For more information: Click here.
56) What is a stream in Scala?
The stream is a lazy list. It evaluates elements only when they are required. This is a feature of Scala. Scala supports lazy computation. It increases the performance of your program.
Example
For more information: Click here.
57) What does Map in Scala Collection?
The map is used to store elements. It stores elements in pairs of key and values. In Scala, you can create a map by using two ways either by using comma separated pairs or by using rocket operator.
Example
For more information: Click here.
58) What does ListMap in Scala?
This class implements immutable maps by using a list-based data structure. You can create empty ListMap either by calling its constructor or using ListMap.empty method. It maintains insertion order and returns ListMap. This collection is suitable for small elements.
Example
For more information: Click here.
59) What is a tuple in Scala?
A tuple is a collection of elements in the ordered form. If there is no element present, it is called an empty tuple. You can use a tuple to store any data. You can store similar type of mixing type data. You can return multiple values by using a tuple in function.
Example
For more information: Click here.
60) What is a singleton object in Scala?
Singleton object is an object which is declared by using object keyword instead by class. No object is required to call methods declared inside a singleton object.
In Scala, there is no static concept. So Scala creates a singleton object to provide an entry point for your program execution.
Example
For more information: Click here.
61) What is a companion object in Scala?
In Scala, when you have a class with the same name as a singleton object, it is called a companion class and the singleton object is called a companion object. The companion class and its companion object both must be defined in the same source file.
Example
For more information: Click here.
62) What are case classes in Scala?
Scala case classes are just regular classes which are immutable by default and decomposable through pattern matching. It uses the equal method to compare instance structurally. It does not use the new keyword to instantiate the object.
Example
For more information: Click here.
63) What is file handling in Scala?
File handling is a mechanism for handling file operations. Scala provides predefined methods to deal with the file. You can create, open, write and read the file. Scala provides a complete package scala.io for file handling.
Example
For more information: Click here.